10 JavaScript Hacks for SEO Developers
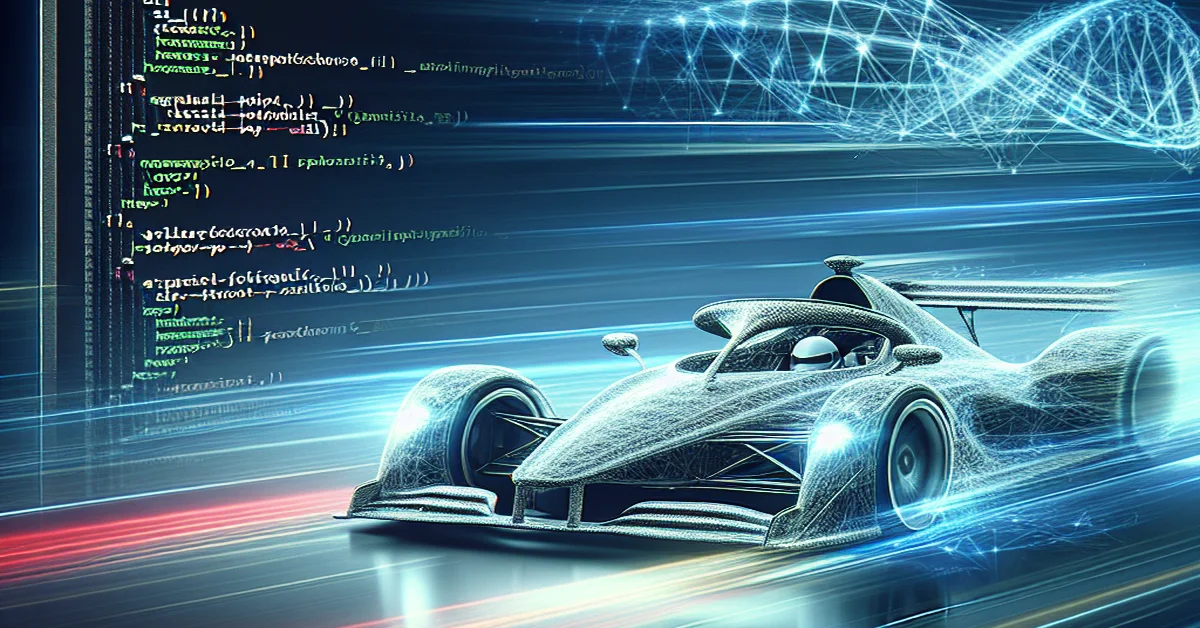
Developers face a challenge: optimizing websites for search engines while improving user experience. With an increasing reliance on JavaScript for dynamic content, understanding its impact on SEO is crucial. Developers can use specific JavaScript techniques to improve search rankings and site performance. These strategies, when implemented correctly, can connect an engaging user interface with search engine visibility.
As search engines improve their ability to crawl JavaScript-heavy sites, developers must adopt tools and techniques thoughtfully. From optimizing image loading to managing redirects, the relationship between JavaScript and SEO is complex and requires a thorough approach. Each choice developers make can affect user engagement and content ranking.
This article explores key JavaScript methods that can boost your SEO strategy. By gaining practical knowledge and actionable insights, you’ll improve your site’s performance and ensure that your efforts reach a wider audience. Let’s dive in.
Table of Contents
- Lazy Loading Images
- Dynamic Rendering Solutions
- Handling JavaScript Redirects
- Implementing Structured Data with JSON-LD
- Optimizing Content Delivery Networks
- Managing Canonical Tags with JavaScript
- Consistent URL Structure with JavaScript
- Implementing Schema Markup Via JavaScript
- Client-Side A/B Testing
- Monitoring Page Performance with Lighthouse
Lazy Loading Images
Lazy loading images boosts your site’s speed, and a faster website is beneficial for everyone. As a developer focused on performance, understanding lazy loading is a valuable skill. It ensures your site only loads images when they become visible, saving bandwidth and decreasing load times.
Understanding Lazy Loading
Lazy loading is an effective technique. For example, if a page has many images, without lazy loading, all those images load when someone visits the page. With lazy loading, images load only as a visitor scrolls down to them.
How to Implement Lazy Loading
You can implement lazy loading in several ways. Modern websites can use HTML’s built-in support for lazy loading with a simple attribute:
<img src="image.jpg" alt="Description" loading="lazy">
This line of code is enough to lazy load an image. When the loading
attribute is set to “lazy,” the browser delays loading images outside the viewport.
Benefits of Lazy Loading
Here are the advantages of lazy loading:
- Reduced Initial Load Time: Only essential resources load initially, speeding up page rendering.
- Bandwidth Efficiency: Only the images that users view are downloaded, conserving bandwidth.
- Improved User Experience: Faster pages keep users engaged, lowering bounce rates.
Advanced Lazy Loading with JavaScript
If the loading="lazy"
attribute isn’t sufficient, perhaps due to older browsers or a need for more control over image loading, JavaScript can help. Libraries like lazysizes provide effective lazy loading with customization options.
Here’s a basic example using plain JavaScript for those wanting a custom solution:
document.addEventListener("DOMContentLoaded", function() {
let lazyImages = [].slice.call(document.querySelectorAll("img.lazy"));
if ("IntersectionObserver" in window) {
let lazyImageObserver = new IntersectionObserver(function(entries, observer) {
entries.forEach(function(entry) {
if (entry.isIntersecting) {
let lazyImage = entry.target;
lazyImage.src = lazyImage.dataset.src;
lazyImage.classList.remove("lazy");
lazyImageObserver.unobserve(lazyImage);
}
});
});
lazyImages.forEach(function(lazyImage) {
lazyImageObserver.observe(lazyImage);
});
} else {
// Fallback for older browsers
lazyImages.forEach(function(lazyImage) {
lazyImage.src = lazyImage.dataset.src;
});
}
});
This script uses the IntersectionObserver
API for efficient image loading. If the observer isn’t supported, it defaults to loading images immediately.
Common Pitfalls and Troubleshooting
Be aware of these common issues:
- SEO Impact: Always include alt text so images contribute to your SEO.
- Image Dimensions: Set width and height attributes to prevent layout shifts.
- Testing: Regularly test lazy loading on various devices and networks to ensure it works smoothly.
Using lazy loading improves your SEO while improving user satisfaction. Your site will perform well, which is vital in today’s web environment.
Dynamic Rendering Solutions
Dynamic rendering is a significant development. Developers know search engines can struggle with JavaScript-heavy sites. Dynamic rendering solutions help improve SEO.
Your website may be visually appealing with many interactive elements powered by JavaScript. Search engines may not “see” or experience your site like users do. They might overlook important content, leading to lost traffic. That’s where dynamic rendering helps.
Dynamic rendering serves a static HTML version of your site to crawlers while providing the fully interactive version to users. Think of it as meeting everyone where they are.
You can implement dynamic rendering in a few ways:
- Server-side rendering (SSR): This generates the complete HTML for each page on the server. Tools like Next.js or Nuxt.js simplify SSR.
- Prerendering: Instead of rendering pages on each request, you render them in advance. Services like Prerender.io can assist with this.
These methods work because providing search engines with straightforward HTML ensures they index your content correctly. Users still receive the dynamic experience with all the JavaScript features.
Setting up isn’t as hard as it seems. Here’s a basic workflow:
1. Detect the user agent on the server.
2. If it’s a search engine bot, serve a pre-rendered HTML snapshot.
3. For everyone else, serve the full dynamic site.
Beyond tools and processes, understanding your site’s essential content is crucial. What must be indexed? Focus your dynamic rendering efforts there to avoid over-engineering your entire site when only parts need this approach.
Some may worry this technique isn’t sustainable or scalable. Technologies progress. Methods like Google’s Dynamic Rendering Guidelines continue to improve, ensuring compliance without sacrificing innovation.
Testing is vital. Run regular checks with Google’s Search Console to see what’s being indexed. Does it match what users see? Use tools like Google’s Mobile-Friendly Test or Bing’s SEO Analyzer to monitor rendering differences.
Dynamic rendering isn’t about manipulating the system. It’s about clarity and opportunity. With these solutions, developers can bridge the gap between design and search visibility. Optimize smart, not hard.
Handling JavaScript Redirects
Handling JavaScript redirects helps maintain SEO value while ensuring users land where they should on your site. Redirects assist both users and search engines in navigating your website, and unfamiliarity with them can lead to headaches. Let’s explore how to handle redirects and their implications for SEO.
Understanding JavaScript Redirects
In web development, server-side redirects (like 301s or 302s) direct traffic from one URL to another. In JavaScript, client-side redirects occur after the page loads, affecting how search engines interpret your site. Although not ideal for SEO, JavaScript redirects can still be useful with some care.
Common Scenarios for JavaScript Redirects
You may encounter situations such as:
- Detecting User Location: Redirect users based on their location to provide relevant content.
- Access Control: Redirect users who do not have permission to access certain pages.
- Mobile Optimization: Direct mobile users to optimized versions of webpages.
Implementing Redirects with JavaScript
When you choose a JavaScript redirect, implementation is straightforward. Here’s a basic approach using JavaScript:
document.addEventListener('DOMContentLoaded', function() {
if (/* some condition */) {
window.location.href = 'https://your-desired-url.com';
}
});
This code listens for the document to fully load before checking a condition to decide whether to redirect.
SEO Considerations
JavaScript redirects can pose challenges for SEO, as search engines may not always interpret them correctly. To reduce potential issues, consider the following:
- Progressive Enhancement: Ensure your core content is accessible as HTML. JavaScript should improve functionality, not replace it.
- Testing with Search Engines: Use tools like Google Search Console to see how search bots render and interpret your JavaScript.
- Minimize Redirect Chains: Each redirect adds an HTTP request, impacting load times and user experience. Keep chains short.
Best Practices for JavaScript Redirects
To make your redirects more SEO-friendly, remember these tips:
- Ensure content loads quickly, reducing reliance on client-side redirects. - Test how various devices handle your redirects for a smooth user experience.
- Document every redirect, especially if it’s JavaScript-based, for easier troubleshooting.
Real-World Impacts
Understanding the real-world effects of managing JavaScript redirects is essential. Poorly handled redirects can dilute SEO impact and frustrate users. For example, unexpected redirect destinations may lead users to exit quickly, increasing your site’s bounce rate. This reflects poorly in search engine algorithms, which prioritize a positive user experience.
While server-side redirects are typically better for SEO, knowing when and how to use JavaScript redirects is valuable for web developers aiming to create dynamic, responsive web experiences. Whether addressing user privacy choices or device-specific needs, JavaScript redirects offer flexibility that, when managed properly, supports usability and visibility.
Implementing Structured Data with JSON-LD
Implementing structured data can feel like deciphering a secret code for SEO gains. It doesn’t have to be a mysterious process, especially with JSON-LD. This format is straightforward for developers comfortable with JavaScript. It’s the choice for embedding structured data on websites and is recommended by search engines like Google.
JSON-LD (JavaScript Object Notation for Linked Data) allows you to add meaning to your web content. Your site’s data is presented in a structured format that search engines understand easily. Think of it as speaking their language, allowing them to better interpret the context of your content.
Why JSON-LD?
Here are some reasons to use JSON-LD:
- Ease of Implementation: JSON-LD scripts don’t interfere with your HTML structure. You can place them in the
<head>
section or just before the closing<body>
tag. - Simple to Edit: JSON-LD data is written as a plain JSON object, making it less prone to errors than HTML markup.
- Asynchronous Loading: JSON-LD can load asynchronously, meaning it doesn’t slow down page speed.
How to Implement JSON-LD
To implement JSON-LD, start by creating a script block, setting the type to application/ld+json
. This is where your structured data will reside.
Here’s a basic example for marking up an article:
<script type="application/ld+json">
{
"@context": "https://schema.org",
"@type": "Article",
"headline": "Boost your SEO skills with these JavaScript hacks",
"description": "An article for developers.",
"author": {
"@type": "Person",
"name": "John Doe"
},
"datePublished": "2023-10-15"
}
</script>
Best Practices
As you apply JSON-LD across your site, keep these practices in mind:
- Keep It Current: Always update your structured data as your content changes.
- Test Your Markup: Use Google’s Structured Data Testing Tool or the Rich Results Test to ensure your JSON-LD code is error-free.
- Stick to Schema.org: This is the most recognized vocabulary, so adhering to it is crucial for getting your rich snippets displayed properly.
Debugging JSON-LD
Troubleshooting is part of a developer’s work. If things don’t work as expected, consider these tips:
- Validate Syntax: JSON is sensitive to typos, so ensure your brackets and commas are in the right places.
- Check for Consistency: Ensure that the data in your JSON-LD matches the visible content on the page.
- Monitor Rich Results: Not every schema type will lead to a rich snippet. Keep an eye on your search results to measure effectiveness.
By implementing JSON-LD effectively, you’ll make it easier for search engines, which can boost your ranking and visibility. This structured approach can uncover rich snippets, improving user click-through rates. Dive in, get to work, and start marking up your pages. It can give your SEO efforts a valuable boost.
Optimizing Content Delivery Networks
Optimizing your Content Delivery Network (CDN) can significantly improve your site’s performance and search ranking. As a developer, you can make smart adjustments to ensure fast content delivery. Here’s how to use JavaScript to improve your CDN.
A CDN is a network of servers that delivers web content to users based on their location, speeding up load times. Faster websites lead to better user experiences, lower bounce rates, and improved SEO rankings. JavaScript is key to optimizing content delivery through these networks.
Implementing CDN Caching with JavaScript
One effective way to optimize your CDN is by using caching mechanisms. Creating JavaScript functions to cache often accessed files can improve load times. You can set cache headers with a service worker. Here’s a basic example:
self.addEventListener('install', event => {
event.waitUntil(
caches.open('my-cache').then(cache => {
return cache.addAll(
'/index.html',
'/styles.css',
'/script.js',
);
})
);
});
Minimize Unused JavaScript
Focus not just on what your CDN delivers, but also on what it doesn’t. Review your JavaScript files to reduce what’s sent over the network. Identify and eliminate unused JavaScript. Tools like Webpack can help optimize and bundle your JavaScript files.
Tips and Tricks
Here are some practical tips to better your CDN’s effectiveness:
- Prioritize Critical Resources: Identify which resources are essential for the initial page load and adjust delivery priorities in your script.
- JavaScript Minification: Reduce the size of JavaScript files to lessen the load.
- HTTP/2 Protocols: Ensure your CDN supports HTTP/2 to use multiplexing and improve loading times.
Monitor and Optimize
Regular monitoring is essential. Use analytics and performance tools to track your CDN’s performance. Tools like Lighthouse can generate reports and pinpoint areas for improvement, including JavaScript issues.
By applying these strategies, your CDN can effectively deliver content. This enhances user experience and boosts your SEO. Remember, small changes can lead to big improvements. Keep experimenting and refining to ensure your site runs smoothly.
Managing Canonical Tags with JavaScript
Managing canonical tags in JavaScript can feel overwhelming. For developers wanting to improve website performance and SEO, understanding this can change the game. Let’s explore how JavaScript can simplify canonical tag management and improve SEO.
Canonical tags help search engines identify the main version of a webpage when similar or duplicate content exists. They’re essential for preventing duplicate content issues. Typically, you’d set canonical tags in the HTML. When generating content dynamically or using a JavaScript framework, it may be necessary to set canonical tags with JavaScript.
Why use JavaScript for this? In a Single Page Application (SPA) or a client-side rendering environment, managing SEO elements like canonical tags dynamically ensures that search engines receive accurate information regardless of how your content is delivered.
Here’s a basic setup:
<head>
<title>Awesome Page</title>
<script>
document.addEventListener('DOMContentLoaded', () => {
const canonical = document.createElement('link');
canonical.rel = 'canonical';
canonical.href = window.location.href.split('?')0;
document.head.appendChild(canonical);
});
</script>
</head>
In the code above, we listen for the ‘DOMContentLoaded’ event to ensure the document is fully loaded before running our script. We create a link
element, set its rel
attribute to ‘canonical’, and use window.location.href
to dynamically get the URL. This automatically removes query strings, leaving the clean URL as the canonical URL. Finally, we append this element to the head
.
Considerations and Best Practices
- Dynamic Pages: For sites with dynamic content, ensure the canonical URL remains the same for those pages, even if pagination or filters change.
- Avoid Duplicates: Make sure no two different canonical URLs point to the same resource, as this can confuse search engines.
- Exploring Further: Using libraries like React, Vue, or Angular? Look into their supported methods for managing head elements dynamically to ensure proper setup.
- Testing is Key: Regularly use tools like Google’s URL Inspection Tool to check that canonical URLs are correctly implemented and indexed as intended.
Troubleshooting Common Issues
Sometimes, even with correctly set canonical tags, search engines may not follow them. Common reasons include:
- Incorrect Tag Placement: Ensure the tag is directly in the HTML
head
. - Multiple Canonical Tags: There should only be one canonical link per page.
- Inconsistent Use Across Similar Pages: Consistency is important to avoid confusing search engines.
Staying Updated
The web constantly evolves. SEO practices change, and what works today might shift tomorrow. Staying informed about the latest practices and algorithm updates is crucial.
Discussing challenges with peers, reading reputable SEO blogs, and participating in developer forums can provide valuable insights.
By managing your canonical tags with JavaScript, you advance your site’s SEO strategy. It can feel challenging, but with the right tools and knowledge, you can steer your site toward SEO success.
Consistent URL Structure with JavaScript
JavaScript can be a tool for establishing and maintaining a consistent URL structure across your website. This is important for SEO because search engine crawlers prefer clean and predictable URLs. You can use JavaScript to keep your URL structure consistent without disrupting the user experience or harming your ranking.
Why URL Consistency Matters
Consistent URLs help users and search engines understand your site’s hierarchy and content. They act like postal addresses for your web pages. When your URLs vary significantly, visitors and crawlers may become lost or confused, impacting your search rankings as search engines struggle to grasp the relevance of your pages.
Creating Consistent URL Structures with JavaScript
For sites built on frameworks like React, Angular, or Vue, JavaScript manages URLs through routing. You can use JavaScript to ensure URL paths are user-friendly and search engine friendly.
For example, with dynamic content loading on the page without refreshing, JavaScript lets you manipulate browser history and URL paths using the history.pushState()
method:
window.history.pushState({}, '', '/new-page-title');
By using this method across your site, you can structure URLs to reflect the specific location or content without triggering a full-page reload, allowing smoother navigation for users and clearer structure for search engines.
Benefits of Consistent URL Structure for SEO
Maintaining a consistent URL structure with JavaScript can:
- Improve user experience by providing clear paths.
- Help search engines index and rank pages better.
- Reduce duplicate content issues, especially on dynamic sites.
Tips for Maintaining URL Consistency
Here are some tips to ensure consistent URLs:
- Always use lowercase letters. URLs are case-sensitive, and lowercase is the web standard.
- Prefer hyphens over underscores for separating words to improve readability.
- Keep URLs short yet descriptive to convey the context or content of a page clearly.
- Avoid unnecessary parameters in URLs. If you need to pass variables (e.g., UTM codes), ensure they don’t disrupt the main structure.
- Redirect old or broken URLs to their new counterparts using 301 redirects to maintain link equity.
Tools to Automate and Check URL Consistency
Proactive monitoring is key. Use tools that alert you to errant URLs or inconsistencies. Consider:
- Google Search Console for checking how your URLs are indexed.
- Ahrefs to find and fix broken links.
- Screaming Frog to crawl your site and identify inconsistencies in real-time.
Setting up a consistent URL structure not only helps users but also signals to search engines your site’s relevance and reliability. As you implement these practices, you’re shaping a roadmap for navigating your digital landscape.
Implementing Schema Markup Via JavaScript
Implementing schema markup through JavaScript may sound technical, but it’s not as difficult as it seems. For developers looking to improve website SEO without using PHP or hardcoding HTML, JavaScript offers a flexible way to embed valuable data, ensuring search engines receive the necessary information without overhauling your existing setup.
Schema markup is a form of microdata that helps search engines understand the context of your webpages. It explains what your data means, not just what it says. This creates rich snippets in search results, potentially increasing click-through rates and visibility. While traditional implementations (usually in HTML) are common, using JavaScript can automate and simplify the process when dealing with dynamic or large-scale content.
Why Choose JavaScript for Schema Markup?
Using JavaScript for schema is especially beneficial when you control the front end but want to avoid complex back-end changes. This method allows you to handle frequent updates and localization changes efficiently. Plus, if JavaScript is your strength, why not make the most of it?
Getting Started with JSON-LD
JSON-LD, or JavaScript Object Notation for Linked Data, is the format recommended by Google for structuring schema markup. Here’s why JSON-LD and JavaScript work well together:
- It fits naturally with JavaScript, as JSON is something every JS developer understands.
- It’s easy to debug and implement.
- You don’t need to edit every HTML file; make changes centrally in your JavaScript files.
Let’s break down the process.
Crafting Your JSON-LD
Creating JSON-LD can be as simple or complex as your data needs. Here’s a basic example of a LocalBusiness
schema:
{
"@context": "http://schema.org",
"@type": "LocalBusiness",
"name": "Your Business Name",
"address": {
"@type": "PostalAddress",
"streetAddress": "123 Main Street",
"addressLocality": "Hometown",
"addressRegion": "ST",
"postalCode": "12345",
"addressCountry": "USA"
}
}
Integrating this into your page is straightforward. Add the following block into your JavaScript file:
const script = document.createElement('script');
script.type = 'application/ld+json';
script.text = JSON.stringify(yourJsonLdObject);
document.head.appendChild(script);
Key Considerations
- Timing: Ensure your JavaScript runs after the page loads. Use DOMContentLoaded or window.onload events.
- Testing: Use the Rich Results Test to validate your markup. This tool checks if you’ve structured the data correctly.
- Crawling: Make sure your server allows search engine crawlers to execute JavaScript. Without this, your efforts might go unnoticed. Test with Google’s Mobile-Friendly Test tool to see how Google renders your page.
Common Pitfalls
Avoid overloading your pages with unnecessary data. Be precise and ensure the information you provide is relevant to your audience. Always keep your structured data updated; stale or incorrect data can mislead search engines and users.
Use JavaScript’s flexibility to make your webpage data-rich and clear for search engines. You’ll improve your site’s performance in SERPs without the hassle of significant back-end changes.
Client-Side A/B Testing
When it comes to client-side A/B testing, a developer’s goal is straightforward: create better user experiences that drive conversions. The tricky part is aligning this with SEO. JavaScript-based A/B testing can become a double-edged sword if not handled carefully. Get it wrong, and you might hurt your search rankings. Get it right, and you boost user engagement while satisfying search engines. Here are some tips.
Understand that JavaScript changes might not be visible to everyone, especially search engines. Google has improved its JavaScript crawling, but it’s not perfect. To ensure A/B testing does not impact your organic traffic, consider server-side testing or hybrid approaches where elements that need to be crawled are delivered on page load while user-specific changes are added with JavaScript.
Having strong user acceptance criteria and using conditionals is essential. Implementing tests with JavaScript provides real-time feedback but also risks diluting important data. Careful client-side A/B testing is crucial:
- Use clear and consistent elements that aren’t drastically different between versions to allow quicker parsing by Google.
- Avoid cloaking — showing different versions to users and search engines can lead to penalties.
- Ensure vital KPIs (CTAs, key messages) are visible in all versions.
When setting up test scenarios, choose JavaScript frameworks that help you manage and serve content variants. Here’s a simple way to implement this:
document.addEventListener('DOMContentLoaded', () => {
let variant = localStorage.getItem('testVariant') 'A';
if (variant === 'A') {
// Implement changes for variant A
document.querySelector('#headline').textContent = "Welcome to Our Sale.";
} else {
// Changes for variant B
document.querySelector('#headline').textContent = "Discover Your Discount.";
}
});
In this code snippet, a JavaScript block determines which content variant a user sees. Using localStorage
, you retain user-specific data without disrupting the SEO-friendly content served initially. This maintains consistency in what crawlers see and preserves your page indexing.
Let’s discuss measuring results. The strength of A/B testing lies in its data-driven approach. Monitor what’s effective for user interaction and for SEO metrics. Are certain versions leading to higher bounce rates? Is one variant causing a drop in search queries?
Evaluate these:
- User engagement metrics (time on page, clicks).
- Search metrics (ranking, impressions).
- Conversion rates (sales, sign-ups).
Lastly, remember caching strategies. Poor caching can serve outdated variants, distorting your data. Make sure your cache logic works with your A/B implementation to provide only the latest versions at all times.
While A/B testing can improve your UX, its effectiveness relies on proper execution. Balanced implementation ensures you are not sacrificing SEO for better user experiences. Continuously iterate, basing decisions on real data to improve your site in a sustainable way.
Monitoring Page Performance with Lighthouse
Boosting your SEO game doesn’t stop at strategizing keywords—it’s about ensuring speed, relevance, and a user-friendly experience. One useful tool is Google’s Lighthouse. This open-source tool analyzes how well your pages are performing and identifies issues that can hurt SEO success.
Think of Lighthouse like a check-up for your website. You run checks not to find problems every time, but to stay ahead. Here’s how you can use Lighthouse to stay on track.
Understanding Metrics
Lighthouse provides important metrics. Understanding these is necessary. You’ll see terms like First Contentful Paint (FCP) or Largest Contentful Paint (LCP). These metrics offer insights into loading performance, which is essential for SEO. Users won’t stay on slow sites, and Google considers speed in its ranking factors. Paying attention to these can help reduce bounce rates.
- FCP: This shows how quickly content begins displaying to users—even a part of it.
- LCP: This metric indicates how long it takes for the largest content element—like an image or a block of text—to be visible.
Both reflect the user’s initial impression. You want these times to be as low as possible.
Uncovering SEO Opportunities
Lighthouse also checks for SEO factors directly. It evaluates whether the document is set up in a search-engine friendly way, including meta
tags, proper indexing, and mobile compatibility. These details can often be overlooked.
Running Lighthouse
To get started with Lighthouse, run it from the Google Chrome browser. Here’s how:
- Open your web page in Chrome.
- Open DevTools using
Ctrl+Shift+I
(orCmd+Option+I
on Mac). -
Click on the “Lighthouse” tab.
-
Choose the categories you want, such as Performance or SEO.
- Click “Generate report” and let it run the tests.
You’ll receive a report highlighting what’s working and what needs improvement.
Taking Action on Recommendations
After you have your Lighthouse report, take action. You’ll find suggestions like optimizing images, removing render-blocking resources, or using browser caching. These changes can improve how your site feels and how it ranks in search engines.
Don’t overlook the details. Metrics like cumulative layout shift
help maintain a stable layout, preventing elements from moving as a page loads. Addressing issues from these reports can significantly improve user experience.
Continuous Monitoring
Monitoring isn’t a one-off task. Websites change constantly. Run Lighthouse periodically to identify new issues that could arise from code or resource updates. Treat it as an ongoing process—each run may reveal new insights and areas to improve.
In SEO, where every millisecond and detail matters, keeping pages optimized with tools like Lighthouse is a smart approach. By focusing on page performance, you align with Google’s ranking signals and provide an experience that keeps users returning. Keep assessing, keep improving, and watch your SEO efforts thrive.
Incorporating JavaScript into your SEO strategy is key for improving website performance. The techniques discussed, from lazy loading images to managing canonical tags, offer solutions for developers looking to optimize their sites. Each hack serves a purpose, helping you create a more efficient user experience while boosting your search engine visibility.
This guide helps you address common SEO challenges. By applying these JavaScript hacks, you’ll improve your skills and make your website more effective. Great SEO isn’t just about driving traffic; it’s about providing value and ensuring a smooth experience for your visitors.