Why Lazyloading JavaScript Is Crucial to SEO
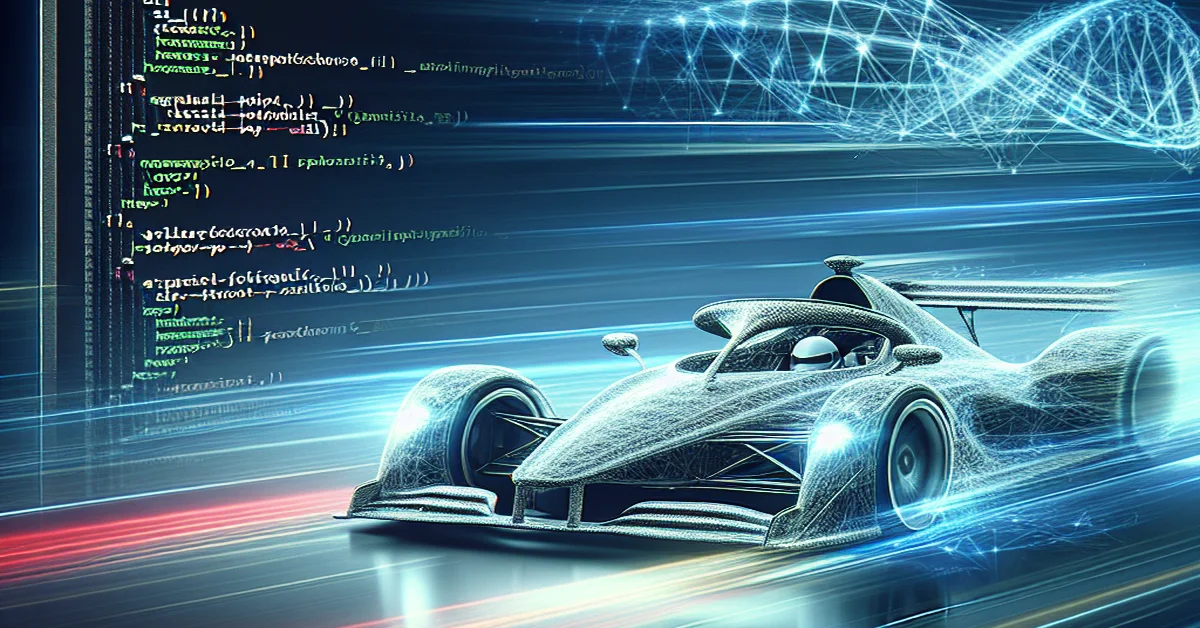
Speed is crucial in web development. Users want smooth experiences, so businesses need to focus on how quickly their sites load. One important area that often gets ignored is JavaScript tags. These pieces of code add interactivity and features, but they can slow down loading times if not handled well. This article looks at lazy loading JavaScript, a method to boost loading speed and enhance user experience..
Websites are getting more complex, making it harder to balance rich content and quick load times. If users get annoyed by slow pages, they may leave before seeing what you offer. This can hurt user satisfaction and your site’s search engine rankings. Knowing how to optimize JavaScript loading is key to keeping users engaged and maintaining visibility.
We’ll explore the benefits of lazy loading JavaScript and practical ways to implement it on different CMS platforms. When used correctly, lazy loading can significantly improve your website’s performance.
Benefits of Lazyloading JavaScript
JavaScript tags play a key role in adding features to a webpage, but if not handled well, they can slow down your site. When visitors load your page, their browsers must download, parse, and execute these tags. This can delay how quickly your content appears. A slow site impacts not speed but also user satisfaction, which can lead to more people leaving without engaging.
When users land on your site and have to wait for JavaScript to load, it’s annoying. Picture someone clicking on a link, waiting around, and then bouncing because they don’t want to deal with the delay. People want quick, smooth experiences. If your site is slow, it’s likely you’ll lose visitors.
The impact goes beyond keeping users around. Search engines like Google take page speed into account when ranking sites. If your pages load slowly, search engines may view your site as less important than others that load faster. You can produce great content, but if users can’t access it quickly, the effort may not pay off.
Why do JavaScript tags slow things down? Here are a few reasons:
- Blocking behavior: When a browser encounters a JavaScript file, it often has to stop rendering other elements on the page until the script loads. This creates a bottleneck, especially with multiple scripts.
- External calls: If your JavaScript pulls in resources from other sites, that adds extra delays. The browser has to request these resources and wait for responses from other servers.
- Large file sizes: Some JavaScript libraries or frameworks are big. Loading them all at once can significantly slow down your page.
To keep your site running smoothly, manage how and when your scripts load. One method is lazy loading JavaScript. This approach delays loading certain scripts until after the main content is visible, improving the user experience. It’s effective for prioritizing content that users can see and interact with right away.
Here are some benefits of managing JavaScript tags well:
- Improved load times: Users access your content more quickly, leading to longer sessions and fewer bounce rates.
- Better SEO performance: As your site’s speed increases, it signals to search engines that your page is efficient, which can help your rankings.
- Enhanced user experience: A responsive site keeps users satisfied and encourages them to stay or return.
To engage your users and provide value, it’s important to consider how JavaScript affects performance. Managing these scripts with lazy loading can lead to significant improvements. The goal is to create a smoother, faster experience that keeps users happy and boosts your search rankings. Understanding these basics will make it easier to implement code examples and strategies across platforms, turning your efforts into real results for you and your audience.
Code Examples for Lazyloading
Lazy loading JavaScript can boost your website’s performance, and it’s easier than you think. Let’s look at practical ways to do this with some code examples.
One simple method to lazy load JavaScript tags is to delay loading until the main content is ready. This way, users see important content without waiting on all scripts. You can add the defer
or async
attributes to the script tag.
Using defer
makes sure the script runs after the HTML document is fully loaded. Here’s how to set it up:
<script src="your-script.js" defer></script>
The async
attribute allows the script to run as soon as it downloads. It doesn’t wait for the HTML to finish loading.
<script src="your-script.js" async></script>
Deciding between these two depends on your needs. If the order of execution is crucial, go with defer
. If not, async
might be the better pick.
For more control, you can create a function to load scripts dynamically after the page has loaded. Here’s a simple example:
function loadScript(url) {
Const script = document.createElement("script");
Script.src = url;
Script.async = true;
Document.head.appendChild(script);
}
// Call this function when you need it.
Window.onload = function() {
LoadScript("your-script.js");
};
This approach lets you load scripts based on user actions, like scrolling or clicking a button.
If you want to load scripts based on events, the Intersection Observer API can help. It tracks whether a user has scrolled to a specific part of the page. Here’s an example:
// Target the script you want to lazy load
const targetScript = "your-script.js";
Const scriptObserver = new IntersectionObserver((entries) => {
Entries.forEach(entry => {
If (entry.isIntersecting) {
LoadScript(targetScript);
ScriptObserver.unobserve(entry.target);
}
});
});
// Use a [placeholder element](https://developer.mozilla.org/en-US/docs/Web/HTML/Attributes/placeholder) to trigger loading
const loadTrigger = document.getElementById("load-trigger");
scriptObserver.observe(loadTrigger);
In this case, when the user scrolls to the placeholder element with the ID “load-trigger,” the script loads. This keeps your initial load light and improves user experience.
If you’re using a CMS, lazy loading might look different. Here’s a quick reference for popular platforms:
- Homegrown Site:
-
Use the methods mentioned above.
- WordPress:
-
Try plugins like “WP Rocket” for effective lazy loading.
- Webflow:
-
Add custom code in the settings to manage script loading.
- Squarespace:
- You can insert code in the Code Injection section to ad how scripts load.
By using these techniques, you can enhance your site’s performance and maintain a good user experience. Tailor these methods to fit your needs, and you’ll end up with a faster, more responsive website.
Lazyloading JavaScript on CMS Platforms
Lazy loading JavaScript on different CMS platforms can greatly improve your website’s performance. Here’s how to implement it on systems like homegrown setups, WordPress, Webflow, and Squarespace.
If you’ve built your own CMS, adding lazy loading is straightforward. A common way is to delay loading JavaScript until the main content has loaded.
Here’s a simple approach:
- Use the
defer
attribute: This tells the browser to download the script while parsing the HTML but to run it afterward.
<script src="your-script.js" defer></script>
- Load scripts on scroll: You can set up an event listener to load scripts when the user scrolls down:
window.addEventListener('scroll', function() {
Const script = document.createElement('script');
Script.src = "your-script.js";
Document.body.appendChild(script);
});
- Intersection Observer: For a more advanced option, use the Intersection Observer API to lazy load your scripts based on visibility.
const loadScript = (src) => {
Const script = document.createElement('script');
Script.src = src;
Document.body.appendChild(script);
};
Const observer = new IntersectionObserver((entries) => {
Entries.forEach(entry => {
If (entry.isIntersecting) {
LoadScript('your-script.js');
Observer.unobserve(entry.target);
}
});
});
Const trigger = document.querySelector('#lazy-load-trigger');
observer.observe(trigger);
In WordPress, you can lazy load JavaScript through plugins or by modifying your theme’s functions.php file.
-
Using a plugin: Plugins like WP Rocket or Async JavaScript let you easily lazy load JavaScript. Just install, activate, and follow the instructions.
-
Manually in functions.php: If you’d rather do it manually, add this code to your theme’s functions.php file:
function add_defer_attribute($tag, $handle) {
If ('your-script-handle' === $handle) {
Return str_replace('src=', 'defer src=', $tag);
}
Return $tag;
}
add_filter('script_loader_tag', 'add_defer_attribute', 10, 2);
Webflow makes script management simple.
- Custom Code settings: Add scripts in the Page Settings or Site Settings. To defer loading, place your script in the Footer code section with the defer attribute.
<script src="your-script.js" defer></script>
- Using Page Load Interactions: You can create an interaction that triggers when the page loads or when a specific element scrolls into view. This helps control when scripts load.
With Squarespace, you also have options.
- Code Injection: Go to Settings > Advanced > Code Injection. You can add your script with the
defer
attribute in the Footer section.
<script src="your-script.js" defer></script>
- Use the Squarespace Code Block: Insert a Code Block on a specific page for your JavaScript. Just make sure to wrap the script in the defer method as shown.
No matter which platform you use, lazy loading JavaScript is about figuring out how and when to load your scripts for better performance. Each method can be customized, so don’t hesitate to try different options. Getting it right makes for a smoother experience for your users.
Lazy loading JavaScript tags can boost your page speed and improve your SEO. This makes your site more user-friendly. By deferring non-essential scripts, your main content loads faster. This helps both visitors and search engines. The code examples and tips in this article will help you implement lazy loading, whether you have a simple site or a complex management system.
Learning to lazy load JavaScript effectively can optimize your site’s performance. These strategies can speed up your pages and enhance your SEO. Use the insights here to take steps that improve your web presence, ensuring a smooth experience for your users.